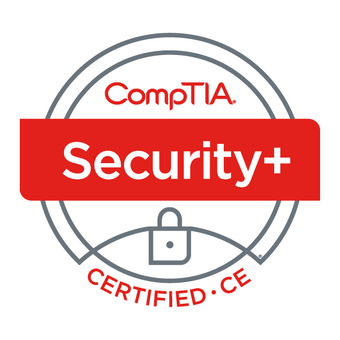
Comptia Security+.
In Massachusetts it’s possible to request a vanity license plate for your car, with your choice of letters and numbers instead of random ones.
Requirements
In plates.py, implement a program that prompts the user for a vanity plate and then output Valid if meets all of the requirements or Invalid if it does not. Assume that any letters in the user’s input will be uppercase.
Structure your program per the below, wherein is_valid returns True if s meets all requirements and False if it does not. Assume that s will be a str. You’re welcome to implement additional functions for is_valid to call (e.g., one function per requirement).
def main():
plate = input("Plate: ")
if is_valid(plate):
print("Valid")
else:
print("Invalid")
def is_valid(s):
... # We need to complete this Function
main()
# Lets get to it..
def main():
plate = input("Plate: ")
if is_valid(plate):
print("Valid")
else:
print("Invalid")
def is_valid(s): # Lets complete the Function "is_valid()"
if len(s) >= 2 and len(s) <= 6: # Input between 2 and 6 characters
if s.isalpha(): # If input is all Letters (no numbers)...
return True # We return True
# If its not all letter (isalpha = False) we jump to the Elif statement..
# We check that input is either a Letter OR a Number (no special chars).
# ..we will also check that the FIRST 2 Chars are Letters (not numbers).
elif s.isalnum() and s[0:2].isalpha(): # If both statements are True, run For Loop. Otherwise return False (below)
for char in s: #Check each Char in the String..
#Check whether Number is in the middle..
if char.isdigit(): #if the char is a number
position = s.index(char) #find the position of the number within the String
if s[position:].isdigit() and int(char) !=0: #Check the Char AFTER the Number. It the next Char is a letter then the Number is in the middle". Ensure its not a zero
#note the : (colon) in the line above. This means "Everything right of the current Char"
return True #If evertyhing has been Correct this far ..return True" i.e The Plate is Vaild
else:
return False #Otherwise return Fasle; the Plate is Invalid!"
main()